People who think that SLICK does not allow zoom movements.
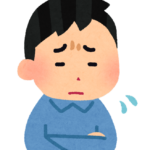
I see a fade in the slick options, but no zoom.
Is there any way to zoom & fade inSlick?
I use slick all the time, so it would be nice to be able to do the zoom movement with slick.
In this issue, we answer these concerns.
Since there is no zoom option in SLICK, many people think that zooming is not possible, but it can be implemented by combining it withtransform: scale
, etc.
What happens when you read this article
- Be able to implement zoom & fade movements using slick.js
- Expand the range of expression of slick
- Be able to think flexibly about “maybe it’s possible to do a move that isn’t in the slick options? You will be able to think flexibly.
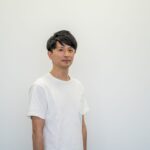
Hello, I am a front-end engineer at a web production company.
I am a front-end engineer at a web production company, coding web pages on a daily basis.
I recently had the opportunity to implement a zoom & fade movement using slick, so I decided to write an article about it.
Until now, I had assumed that it could not be done because there is no zoom in the slick options, but after a little research, I was able to implement it.
However, I usually use Vegas.js when I want to zoom unless I have a special reason to do so.
Image of the finished product
See the Pen Untitled by shimpei (@shimpei) on CodePen.
Here is an image of the finished product.
Zoom & fade movement is implemented using slick.js.
Mounting Method
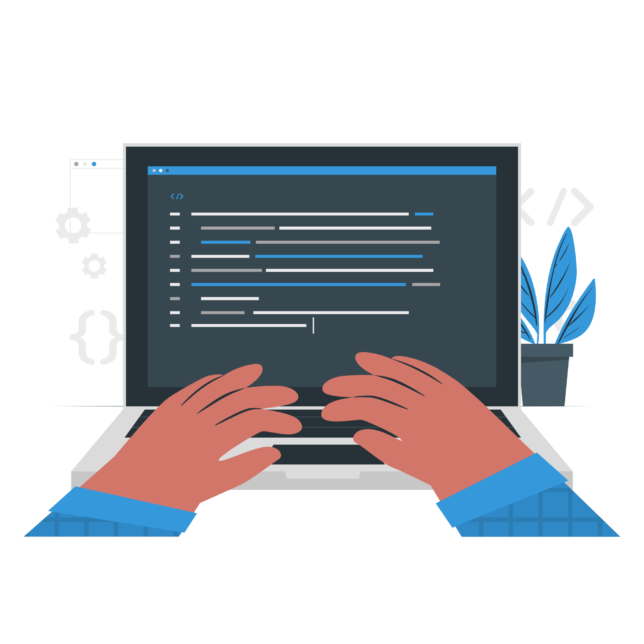
HTML
The HTML looks like this.
It is the same as when slick is used normally.
<div class="slider-wrap">
<div class="slide-item">
<img src="https://picsum.photos/2000/2000" alt="">
</div>
<div class="slide-item">
<img src="https://picsum.photos/1000/1000" alt="">
</div>
<div class="slide-item">
<img src="https://picsum.photos/800/800" alt="">
</div>
</div>
CSS
Next is CSS.
Since there is no zoom in the slick options, we will create the movement on the CSS side.
First, use transform:scale
, which can enlarge an element.Combine transform:scale
and animation (@keyframes
) to create an animation for zooming.
The animation for zooming can be called with the class name .slick-animation (used with jQuery)。
// Size of slider .slider-wrap { height: 500px; width: 80vw; margin: 0 auto; overflow: hidden; } // Image display (e.g. object fitting) .slide-item>img { width: 100%; height: 100%; object-fit: cover; } //Zooming Animation @keyframes fadezoom { 0% { transform: scale(1); } 100% { transform: scale(1.1); } } // With this class name, you can call an animation that zooms .slick-animation { animation: fadezoom 5s 0s forwards; }
jQuery
Finally, jQuery.
//Event that occurs when slick is initialized (Class of .slick-animation is attached to the slide that is initially displayed) $('.slider-wrap').on('init', function () { $('.slick-slide[data-slick-index="0"]').addClass('slick-animation'); }) .slick({ autoplay: true, infinite: true, fade: true, slidesToShow: 1, slidesToScroll: 1, arrows: false, speed: 1000, autoplaySpeed: 3000, pauseOnFocus: false, pauseOnHover: false }) .on({ // Event that occurs before the slide moves beforeChange: function (event, slick, currentSlide, nextSlide) { //Attach a .slick-animation class to the displayed slide $(".slick-slide", this).eq(nextSlide).addClass("slick-animation"); //Later, add a .stop-animation class to erase the .slick-animation class $(".slick-slide", this).eq(currentSlide).addClass("stop-animation"); }, // Event that occurs after the slide is moved afterChange: function () { //Remove animation classes for slides that are not visible. $(".stop-animation", this).removeClass("stop-animation slick-animation"); }, });
All we do is this.
- Attach animation class to the initially displayed slide
- Set slick options (fade, speed, etc.)
- Attach animation class to the displayed slide
- Remove animation class for hidden slides.
In short, we just add and remove animation classes created with CSS.
I have a template for adding and removing classes before and after the animation is displayed, but I did not come up with this on my own.
Event that occurs at initialization [init]
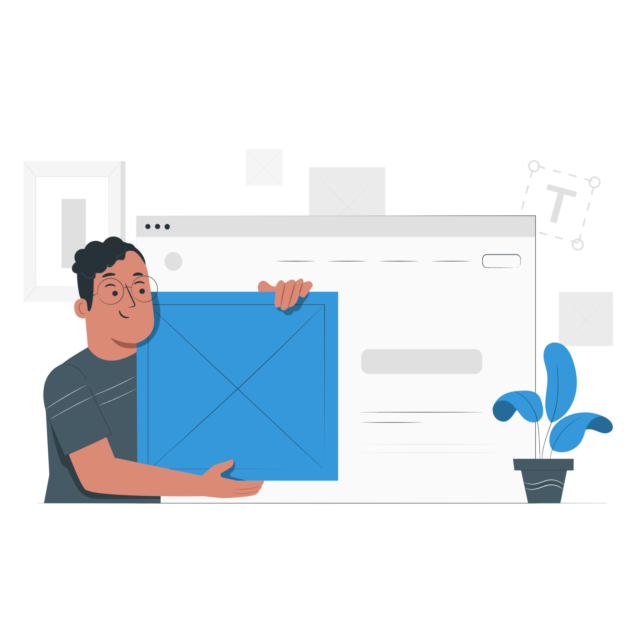
This event must be described first or it will not work. Put it at the top!
To learn more about init, please click on the link below.
The explanation is very detailed.
Event that occurs before the slide moves【beforeChange】
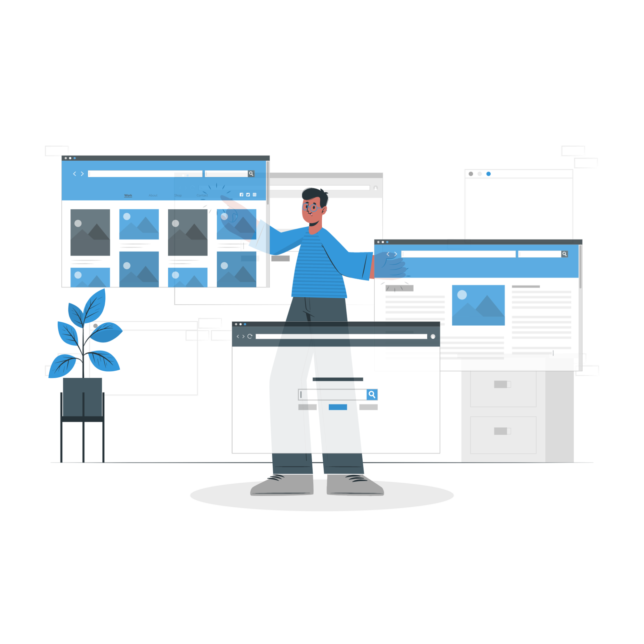
If you write various things in this section, they will be executed before the slide is moved.
Note that nothing happens on the very first slide!
$('.slider').on('beforeChange', function(event, slick, currentSlide, nextSlide){ //Describe here the events before the slide is moved});
Event that occurs after the slide is moved【afterChange】
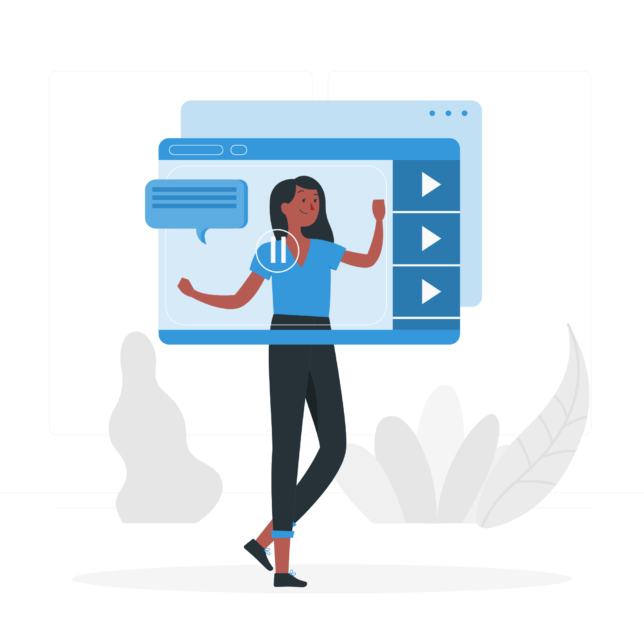
Similarly, if you write various things in this section, they will be executed after the slide is moved.
$('.slider').on('afterChange', function(event, slick, currentSlide){ //Describe the event after the slide is moved here });
That’s all for the jQuery explanation.
Finally
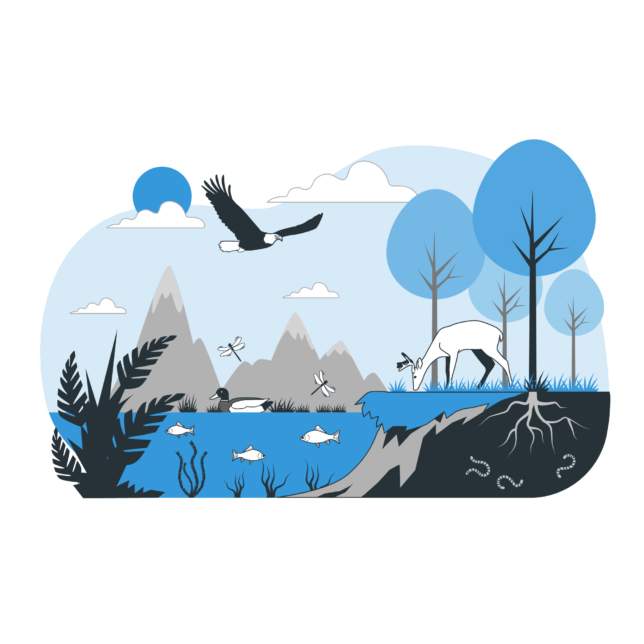
The explanation is a little longer this time, but it can be used by copy and paste, so it should not take that long to implement quickly.
If you know the contents of init
, beforeChange
, and afterChange
, it is possible to display the number of slides and the number of slides.
I would like to deepen my understanding as I use it little by little.
Thank you for the last one!